CSS Art – scale3d() with rotate3d() Transforms
Master CSS scale3d() and resize objects in 3D! Mix it with rotate3d() and create stunning web transformations in this step-by-step article.
Introduction
In this article, you’ll learn how to use the scale3d()
function to manipulate a square in three-dimensional (3D) space.
The scale3d()
function’s property values, including the x-axis, y-axis, and z-axis, affect the square in different ways, and you will learn how to use them through multiple examples. You’ll learn how to combine scale3d()
with rotate3d()
to enhance the square shape’s 3D effect.
CSS functions you’ll explore in the following examples:
scale3d()
rotate3d()
Preview
The first two examples teach how to utilize the scale3d()
function.
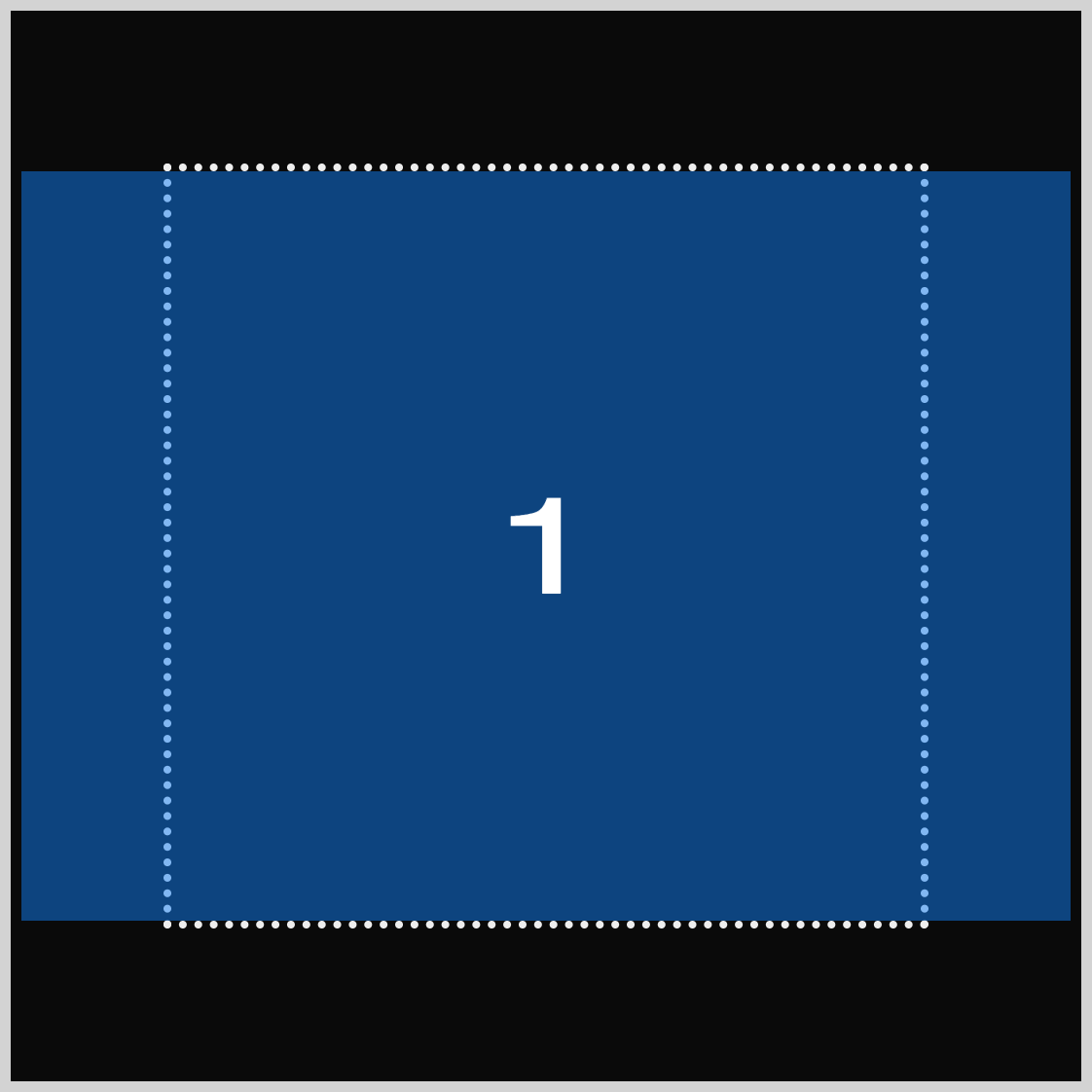
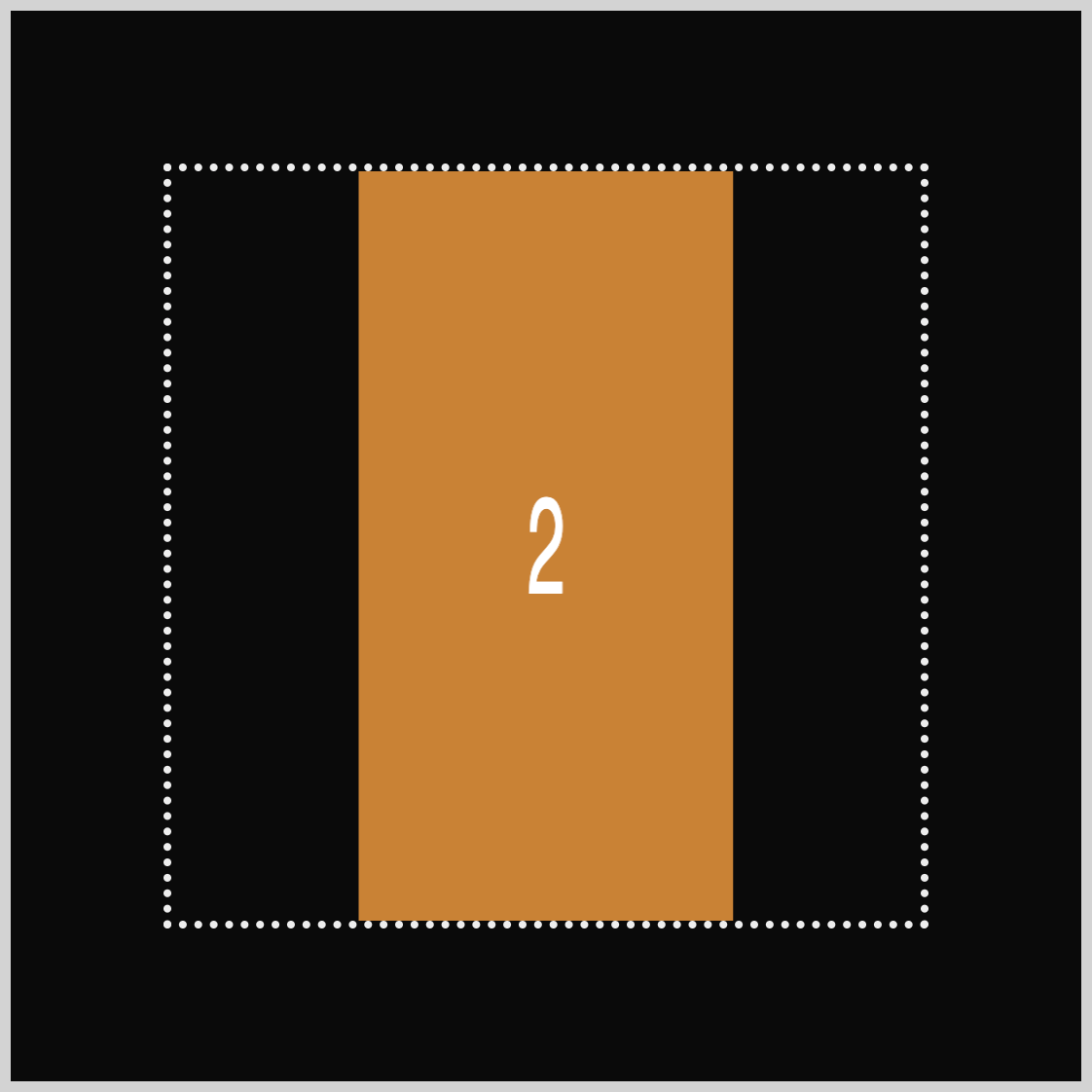
The final two examples integrate scale3d()
with rotate3d()
, demonstrating how to adjust the shape’s rotation and axes for a more three-dimensional appearance.
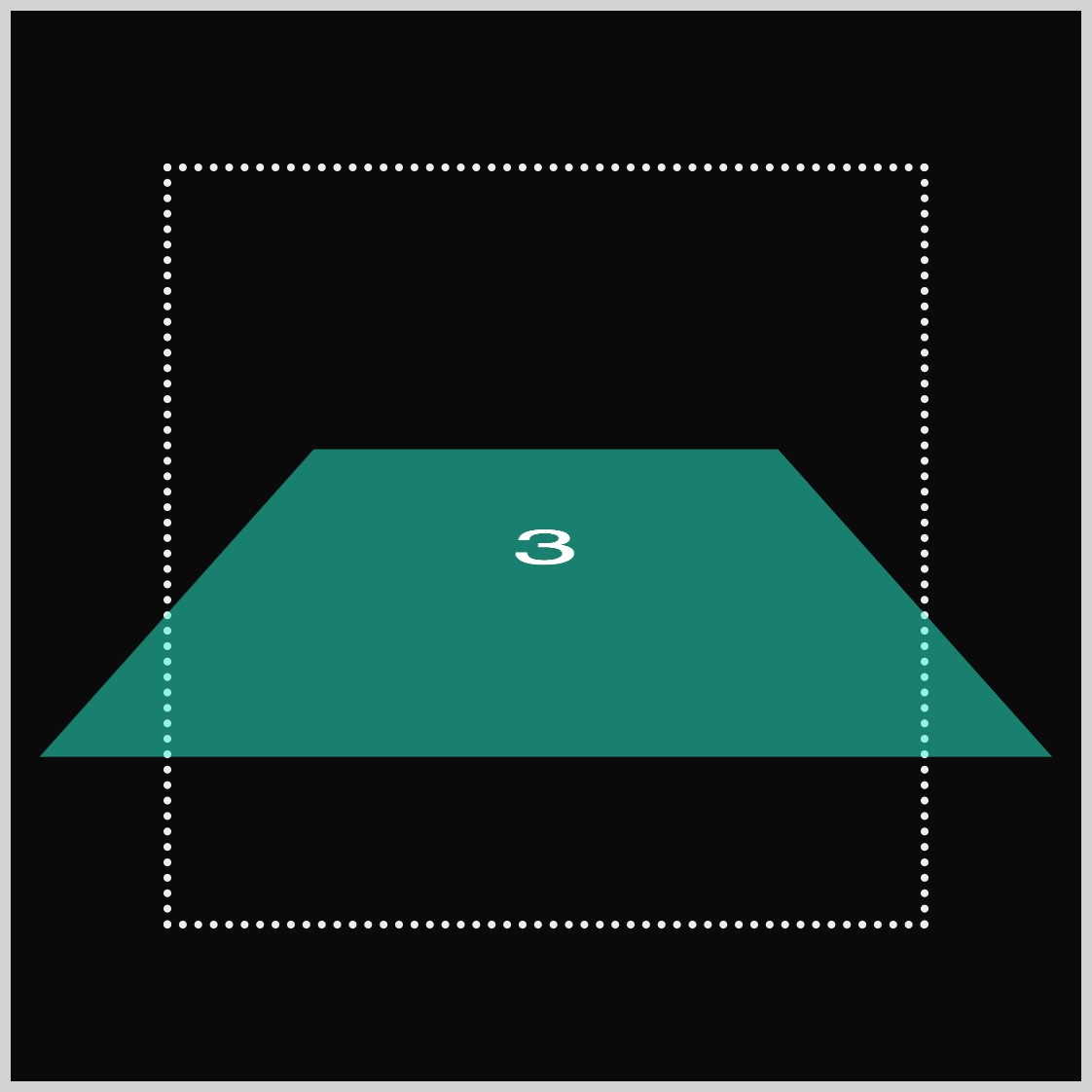
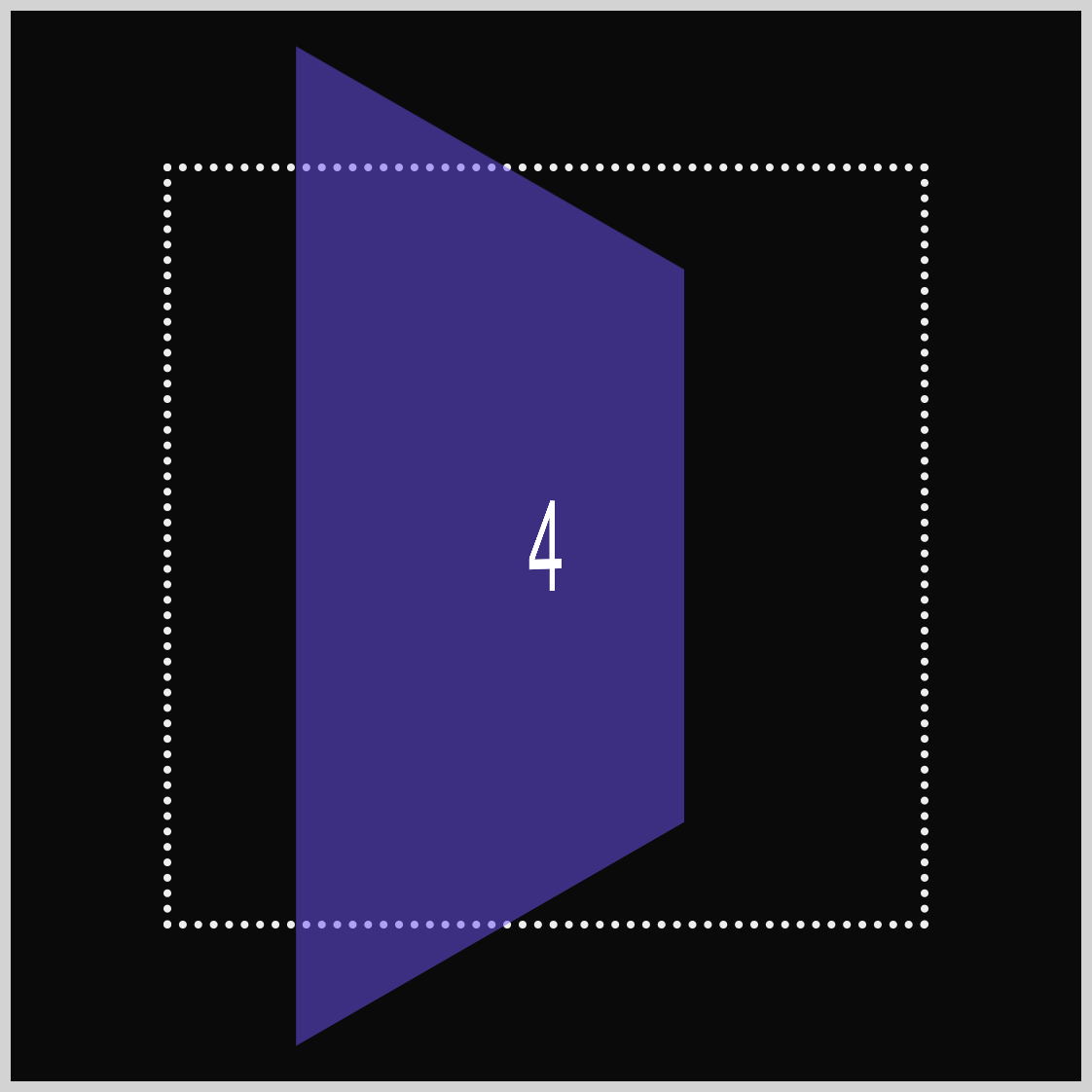
Prerequisites
Essential CSS and HTML knowledge will help you understand the concepts and techniques introduced in this article. Jump over to this article if you require an HTML and CSS primer.
We assume you have configured tools to modify CSS. If not, this article will guide you through the setup process.
HTML Structure
<div class="container">
<div class="shape-container">
<div class="square one">1</div>
</div>
</div>
<div class="container">
<div class="shape-container">
<div class="square two">2</div>
</div>
</div>
<div class="container">
<div class="shape-container">
<div class="square three">3</div>
</div>
</div>
<div class="container">
<div class="shape-container">
<div class="square four">4</div>
</div>
</div>
container
is the outermost enclosure. It enables the content to be centered and draws a light gray border. The rest of the divs represent each image component.
Keep the HTML structure as is for the image to display correctly.
Body and Container Div CSS
CSS code for the body
and container
div.
/* Body and Container Settings */
/* Center shapes */
body {
margin: 0;
padding: 0;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
flex-wrap: wrap;
}
/* Set background and border color */
.container {
min-width: 500px;
height: 500px;
border: 5px solid lightgray;
background: #0a0a0a;
position: relative;
margin: 5px;
display: flex;
justify-content: center;
align-items: center;
}
Shape Container and Square
In this section, you will learn how to make these two images:
- Shape Container
- Square
The images have a parent-child relationship where the Shape Container, shape-container
, encloses the Square, square
.
<!-- Shape Container and Square parent-child -->
<div class="shape-container">
<div class="square one">1</div>
</div>
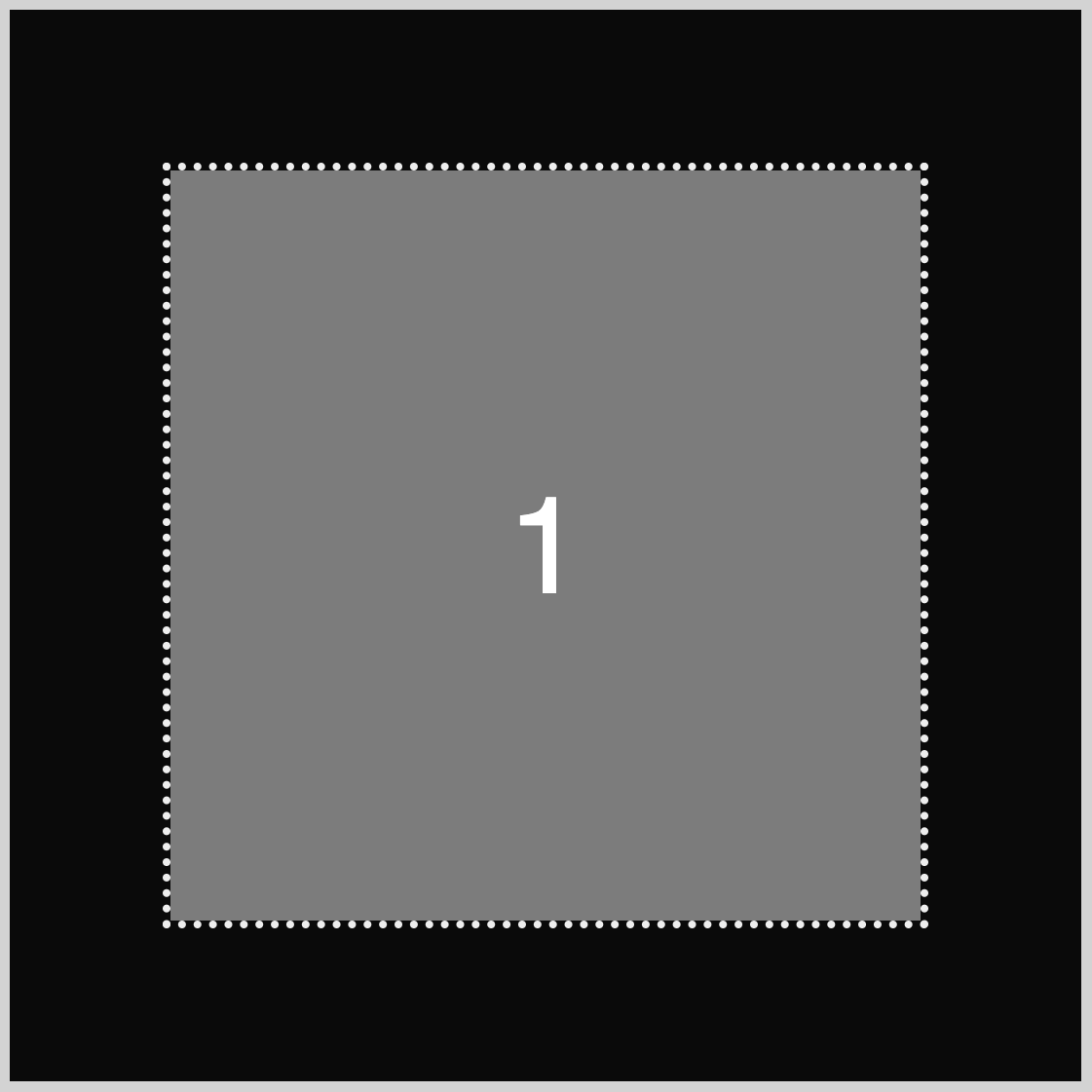
/* Shape Container */
.shape-container {
position: relative;
width: 350px;
height: 350px;
border: 4px dotted #eeeeee;
perspective: 500px;
}
The Shape Container has a width and height of 350 pixels (350px
). It features a four-pixel (4px
) white dotted border. The positioning is defined as relative
, and the perspective property is set to 500 pixels (500px
).
/* Square */
.square {
position: relative;
width: 100%;
height: 100%;
color: white;
font-family: sans-serif;
font-size: 4em;
display: flex;
justify-content: center;
align-items: center;
}
The Square is the same width and height as the Shape Container, defined using the 100%
property value. The font color is white
, the font family is sans-serif
, and the font size is 4em
. Additionally, the display is configured as flex
, with both justify-content and align-items set to center
.
The Square shape does not have a background color. In the upcoming sections, the background color will be specified for each example.
scale3d() CSS Function
The scale3d()
CSS function describes a transformation that alters an element’s size in three-dimensional (3D) space.
Syntax:
scale3d(sx, sy, sz)
sx
: A number representing the horizontal or x-axis of the scaling vector.sy
: A number representing the vertical or y-axis of the scaling vector.sz
: A number representing the depth or z-axis of the scaling vector.
The first example will be the x-axis horizontal scaling.
X-axis Horizontal Scaling
The initial example demonstrates how to scale the square along its horizontal or x-axis.
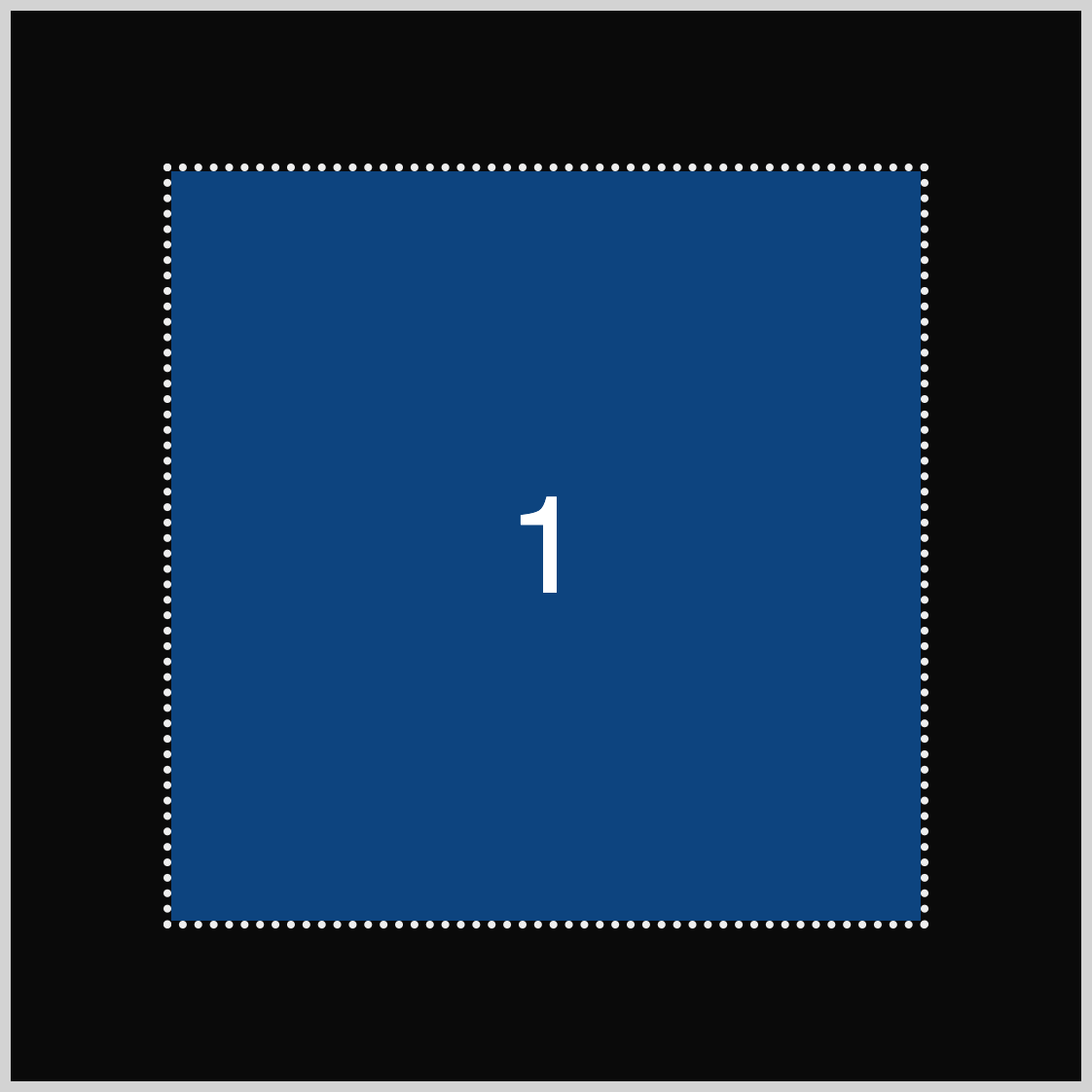
The blue square begins inside the shape container, then scales horizontally along its x-axis, and stops just before the gray border.
.square.one {
background: #0d7df57f;
transform: scale3d(1.4, 1, 1);
}
- The
background
property value is set to#0d7df57f
, a blue shade with 50% opacity. - The
scale3d(1.4, 1, 1)
function assigns a value of1.4
to the x-axis and1
to both the y and z-axes. This change increases the horizontal value of the square shape by about forty percent (.4
) compared to its default width property value.
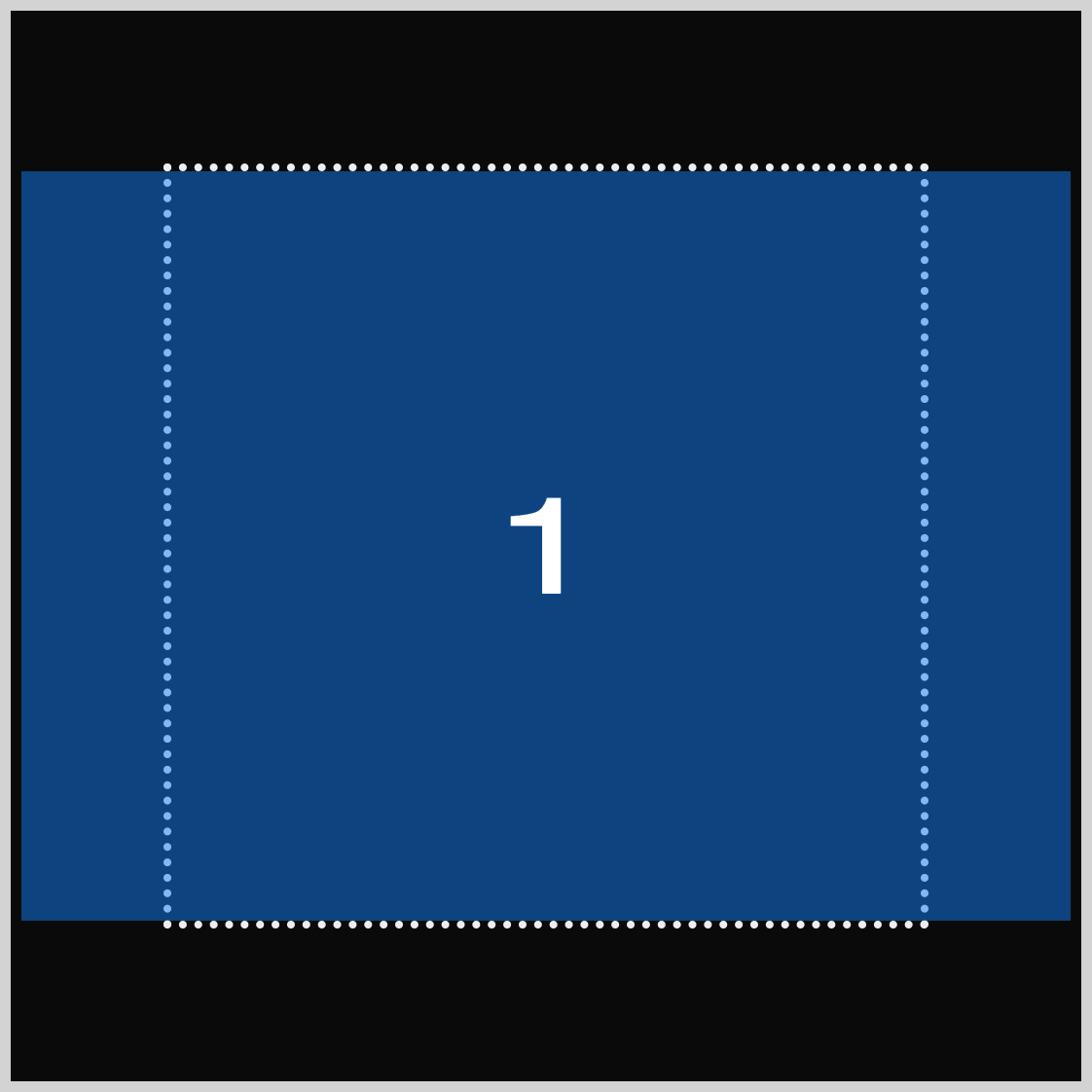
Next, let’s try vertical y-axis scaling.
Y-axis Vertical Scaling
The second example demonstrates vertical (y-axis) scaling. When combined with horizontal scaling, this transforms the square into a vertically elongated rectangle.
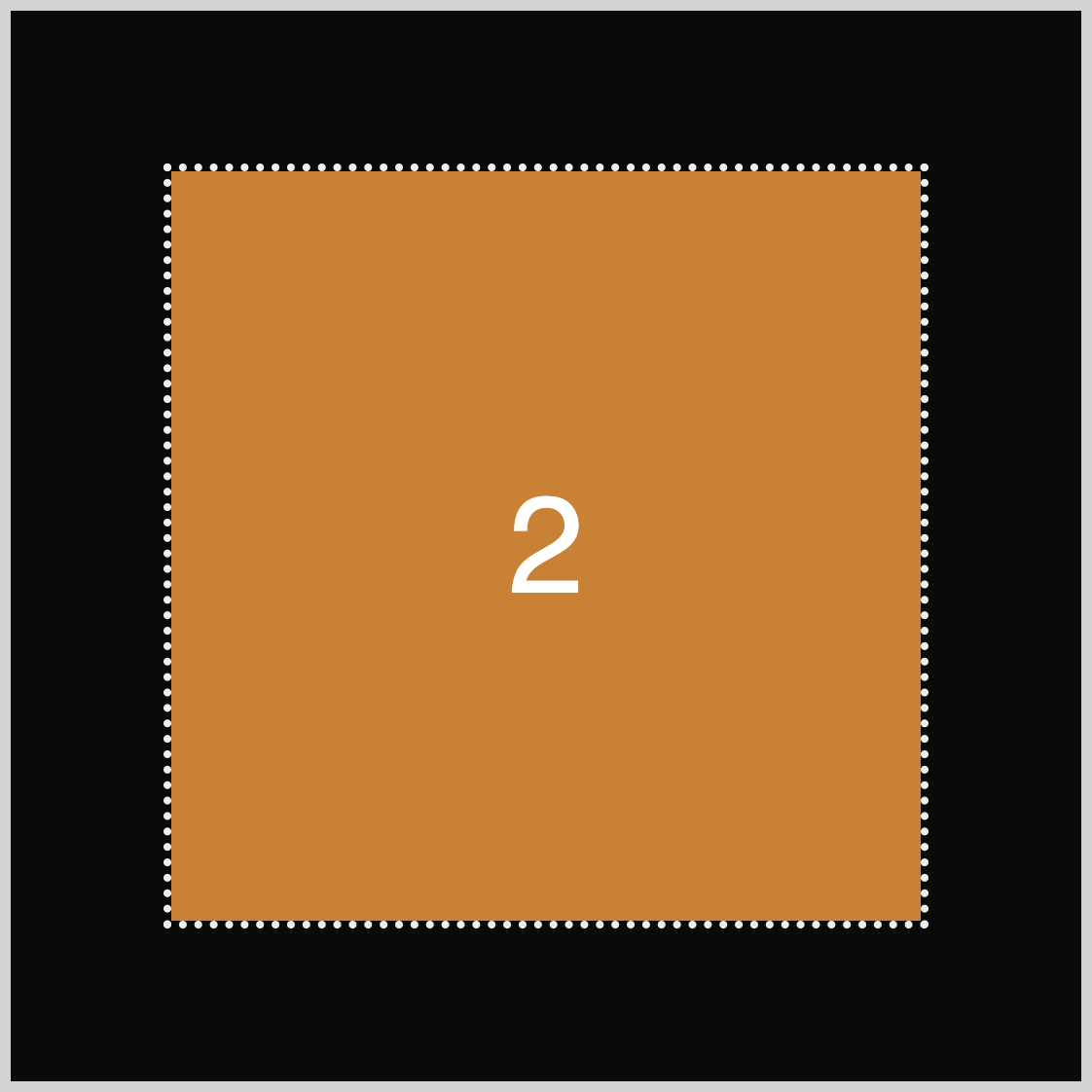
.square.two {
background: #f99f3ecc;
transform: scale3d(0.5, 1.3, 1);
}
The background color is set to #f99f3ecc
, featuring an orange hue with 50% transparency. The transformation scale3d(0.5, 1.3, 1)
can be broken down as follows: the x-axis is scaled to 0.5
, reducing the square’s width to 50% of its original width. For the y-axis, a value of 1.3
increases the height of the square by roughly 30% from its original size.
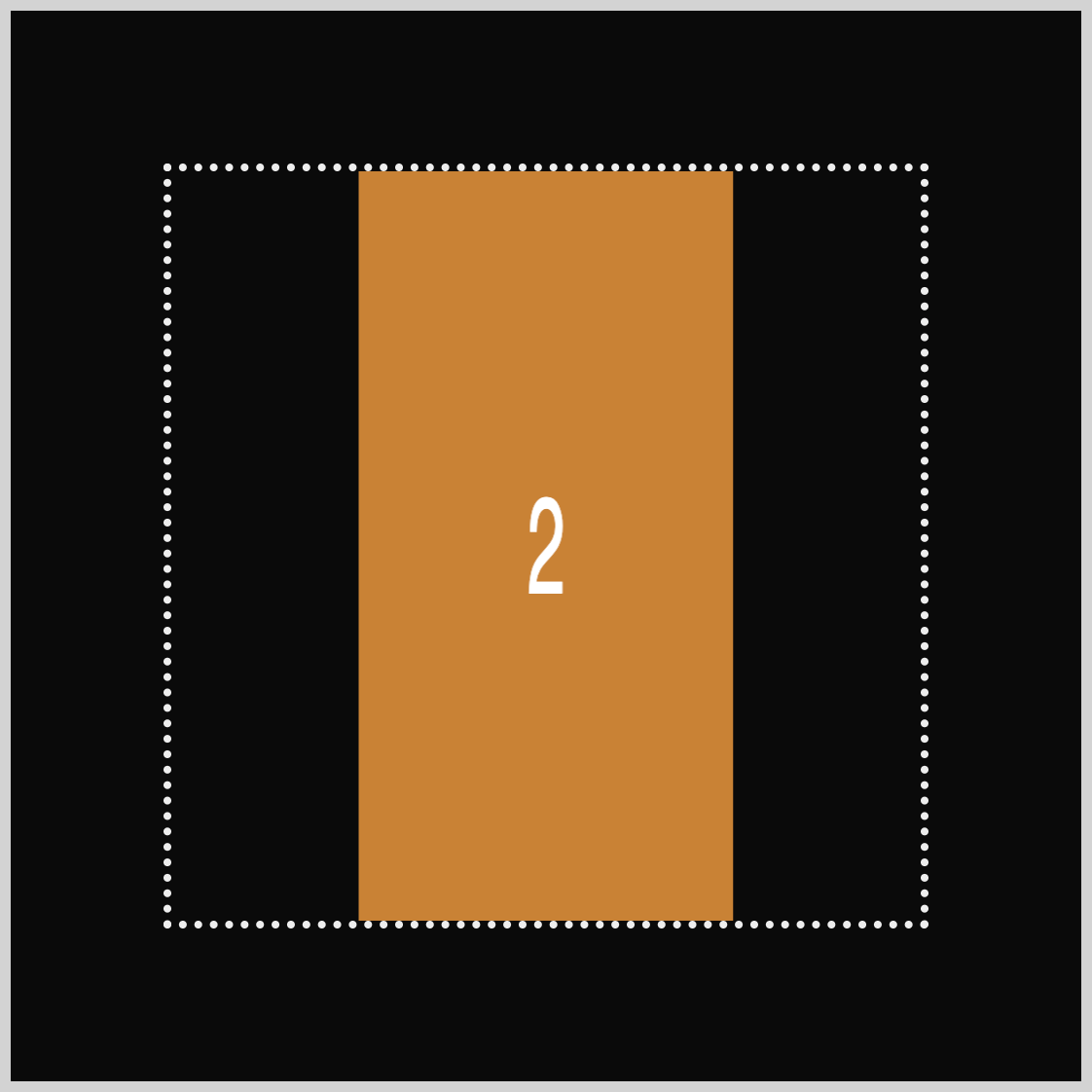
By reducing the x-axis property value to 0.5
, the square is scaled to 50% of its original width.
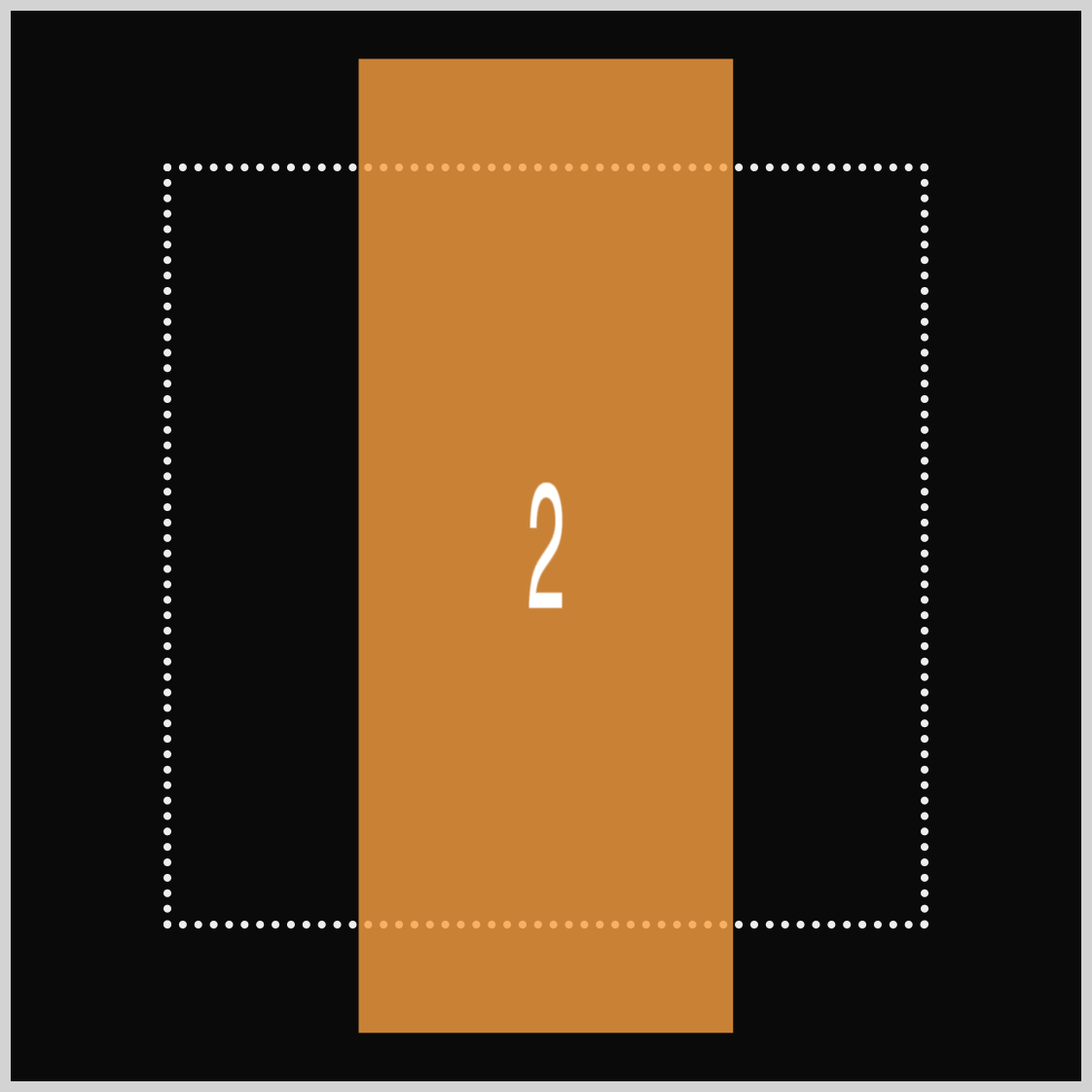
The square goes over its original height when you apply 1.3
as its y-axis property value.
For the third example, you’ll learn how to scale the depth or z-axis.
Z-axis Depth Scaling with Rotation
In this section, you’ll combine scaling and rotation, using rotate3d()
to create a sense of depth. Refer to this article for details on using rotate3d()
.
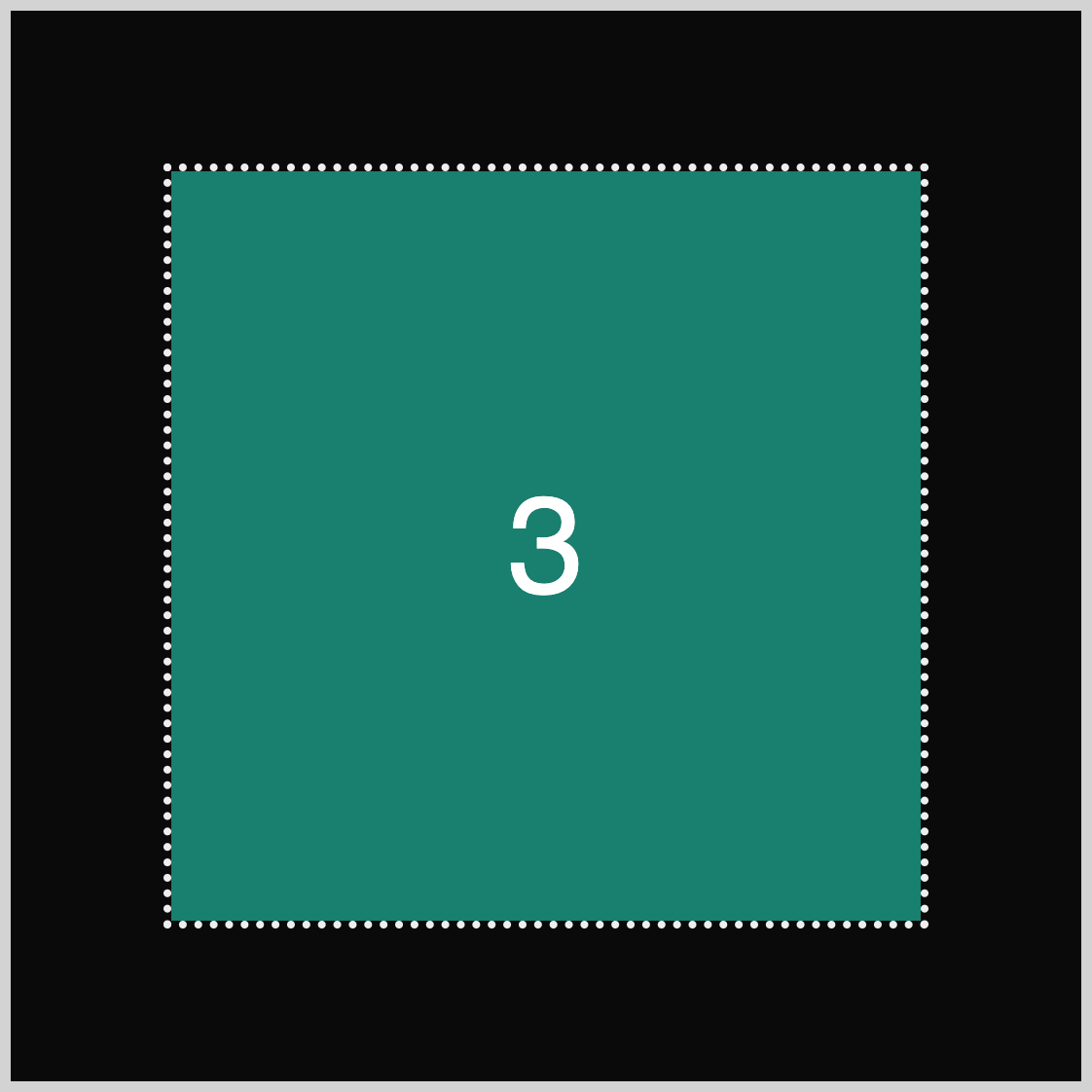
First, you’ll set the width and then the height property value. Next, you modify the depth property value and configure the x-axis value in the rotate3d()
function to complete the third example.
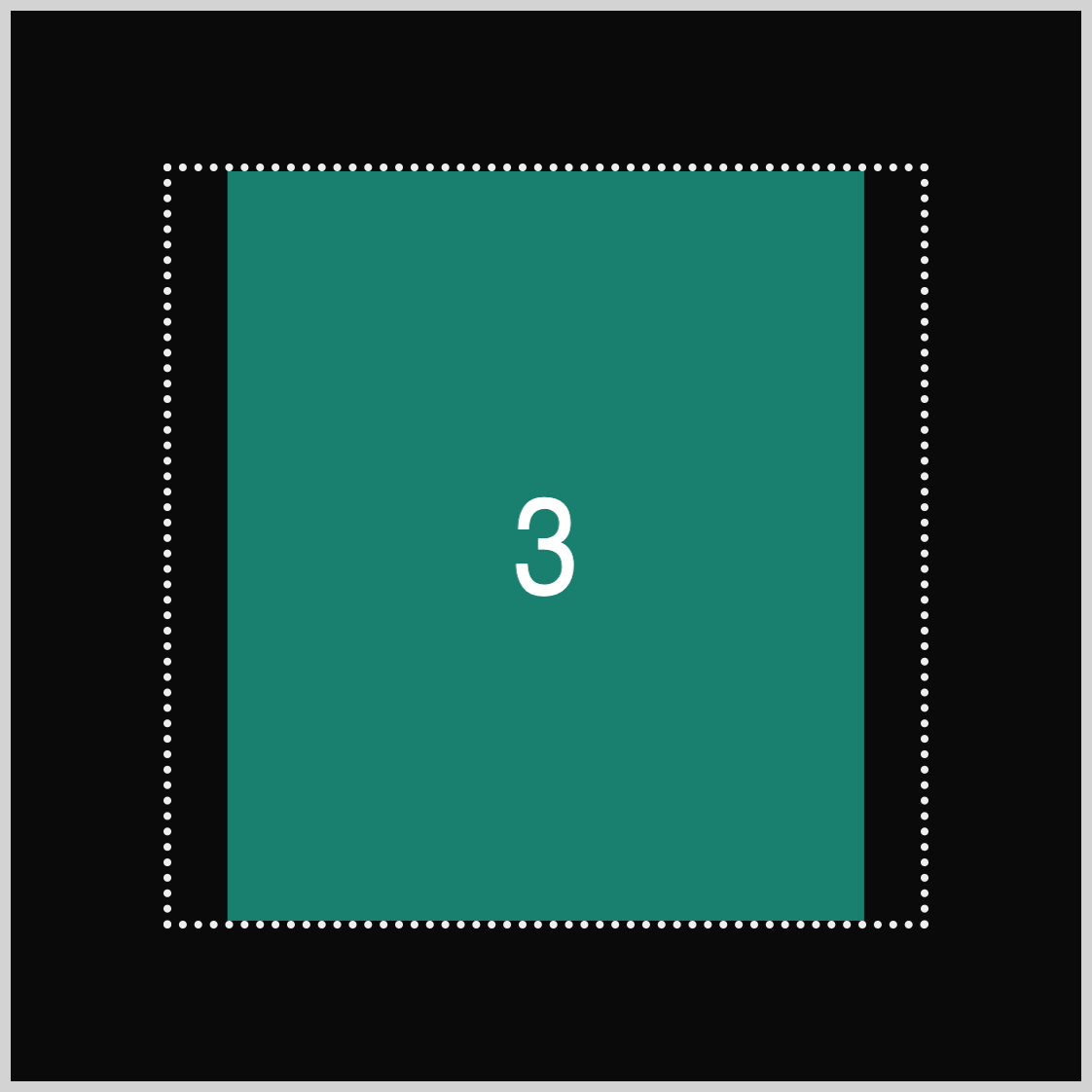
.square.three {
background: #0df5d37f;
transform: scale3d(0.85, 0.5, 1.5) rotate3d(1, 0, 0, 45deg);
}
The CSS properties mentioned above target both the .square
and .three
selectors. The background color is #0df5d37f
, which represents a green hue with 50% transparency.
scale3d(0.85, 0.5, 1.5)
- The horizontal (first function value) is set to
0.85
, scaling the square to 85% of its original width. - Setting the second function value to
0.5
reduces the square’s height to 50%. - Using
1.5
as the third function value increases the square’s depth by 50% compared to its original position.
rotate3d(1, 0, 0, 45deg)
- A value of
1
for the first function indicates that the square will rotate horizontally around its x-axis. 45deg
will rotate the square by 45 degrees.
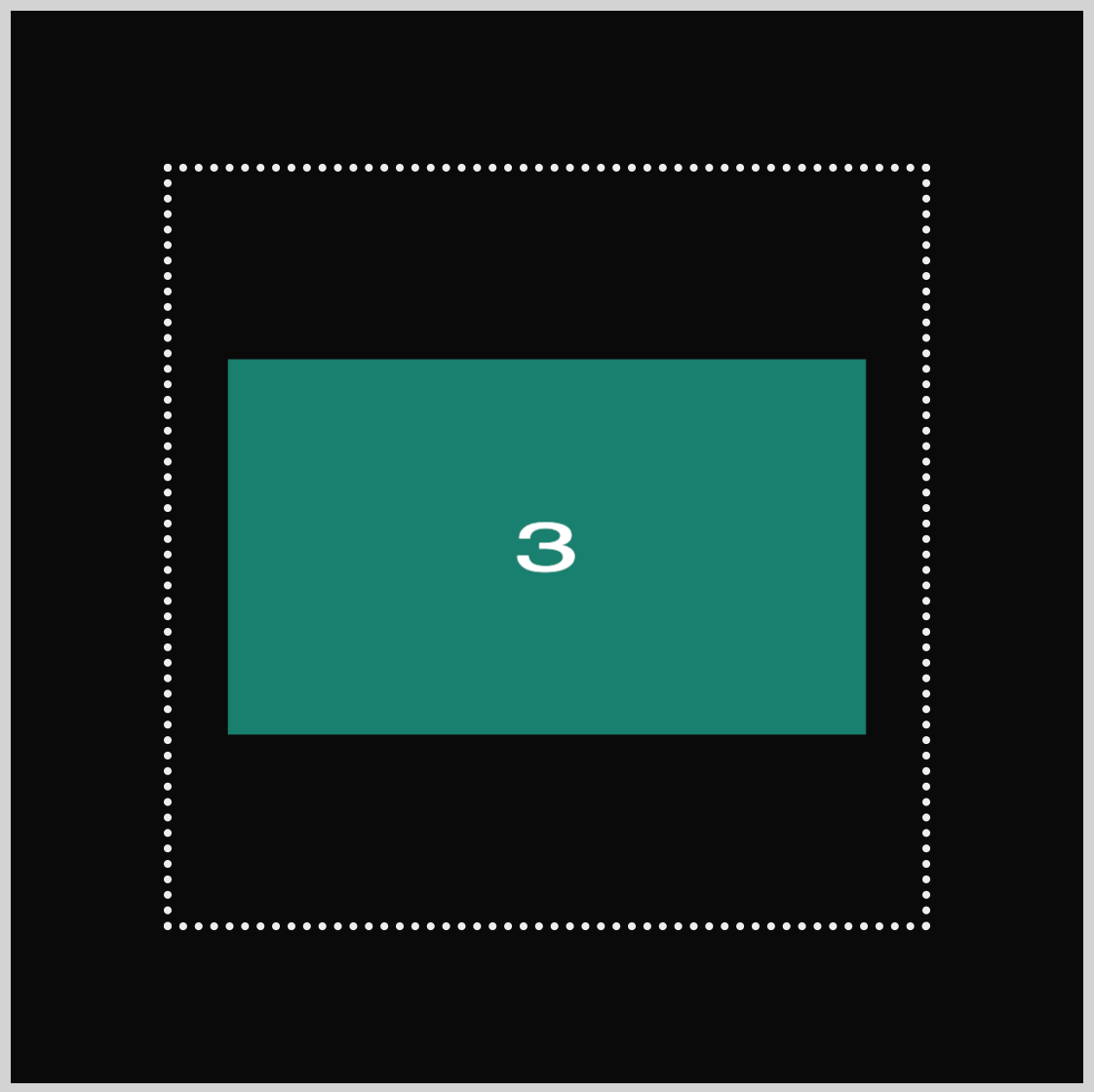
At this stage, rotate3d()
has not yet been applied to the square shape.
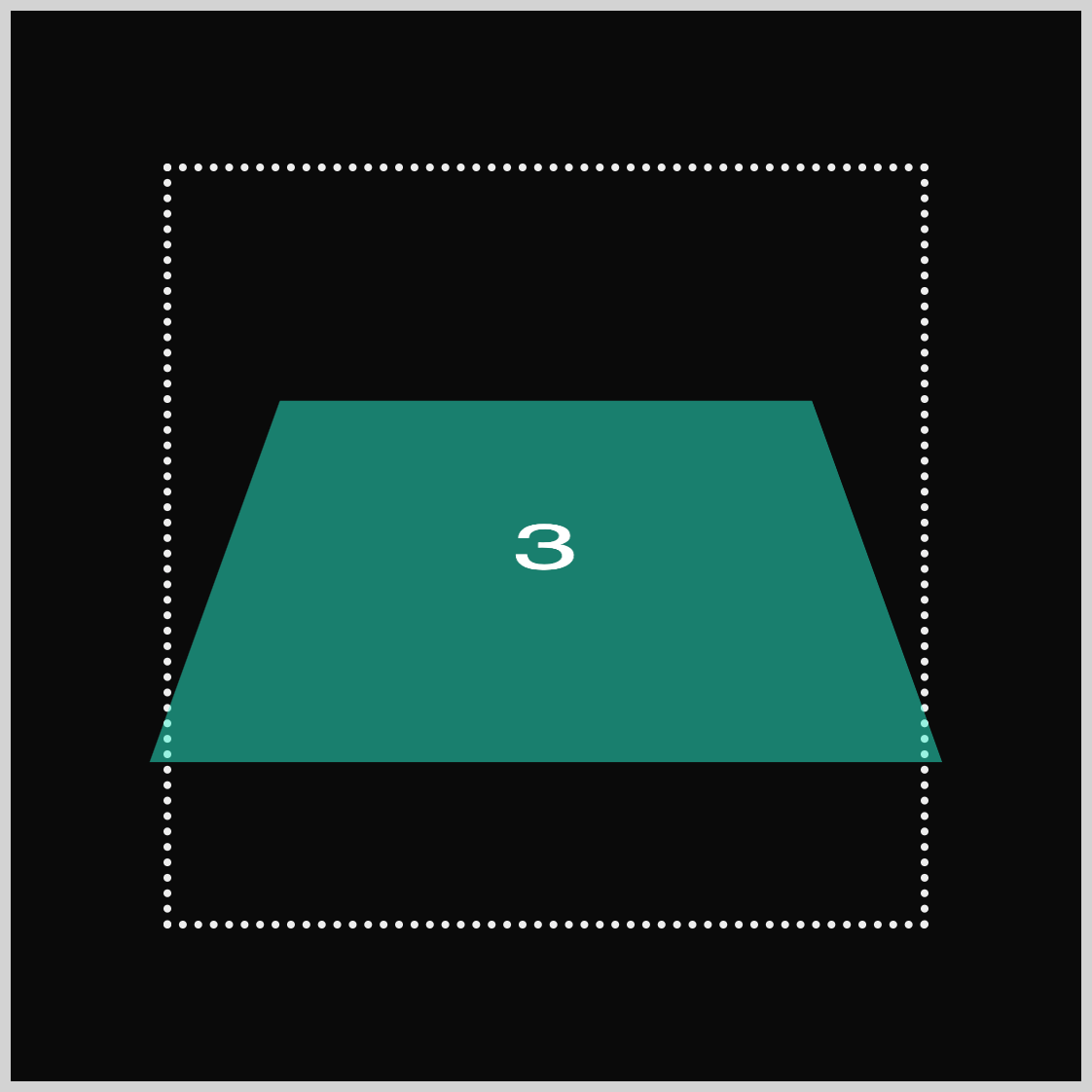
The scale3d()
depth, or z-axis, is set to 0.5
at this stage, and rotate3d(1, 0, 0, 45deg)
has been applied to the square shape.
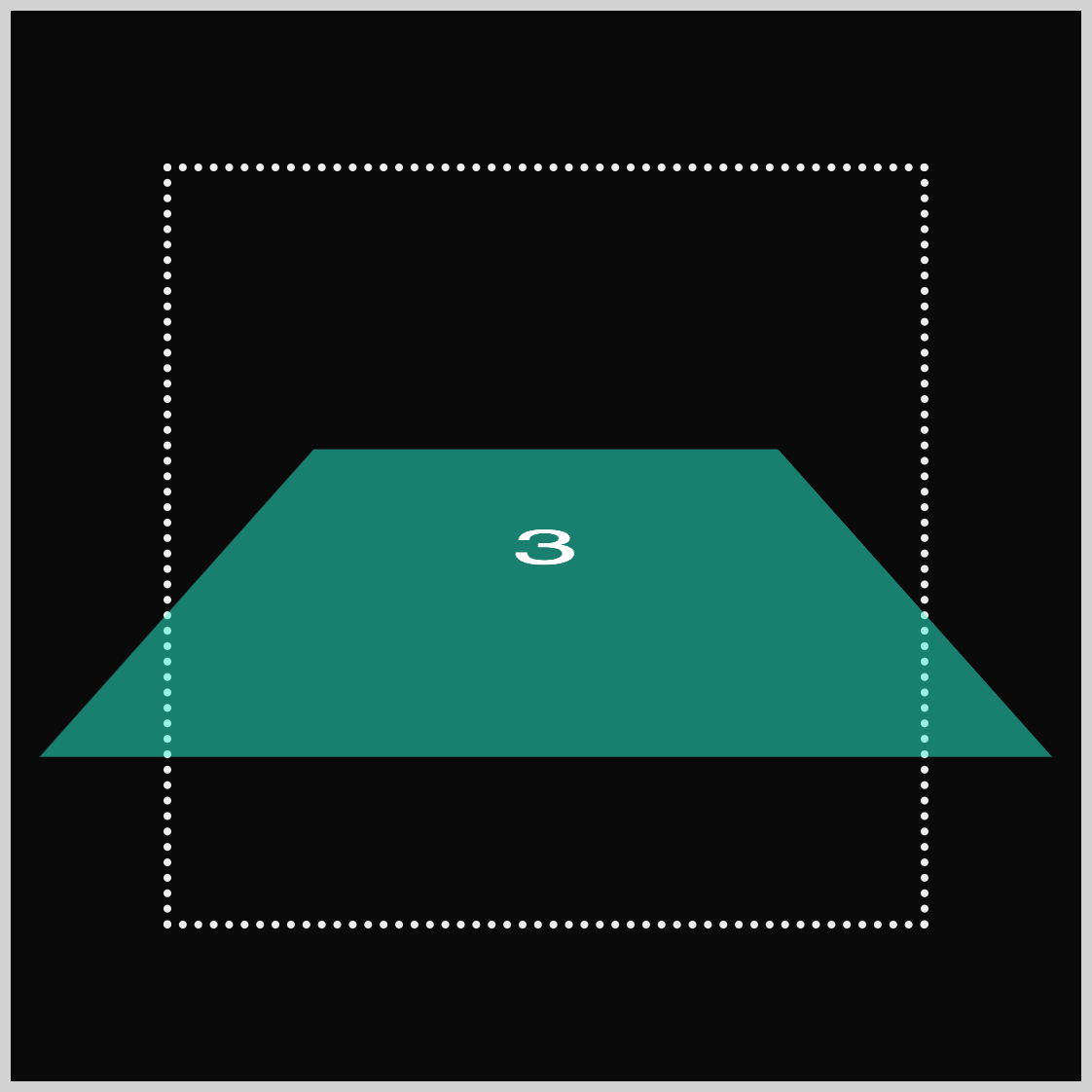
Both the scale3d()
and rotate3d()
function values are applied, producing the square end shape.
For the final section, let’s work on another three-dimensional (3D) example.
3D Scaling and Rotation
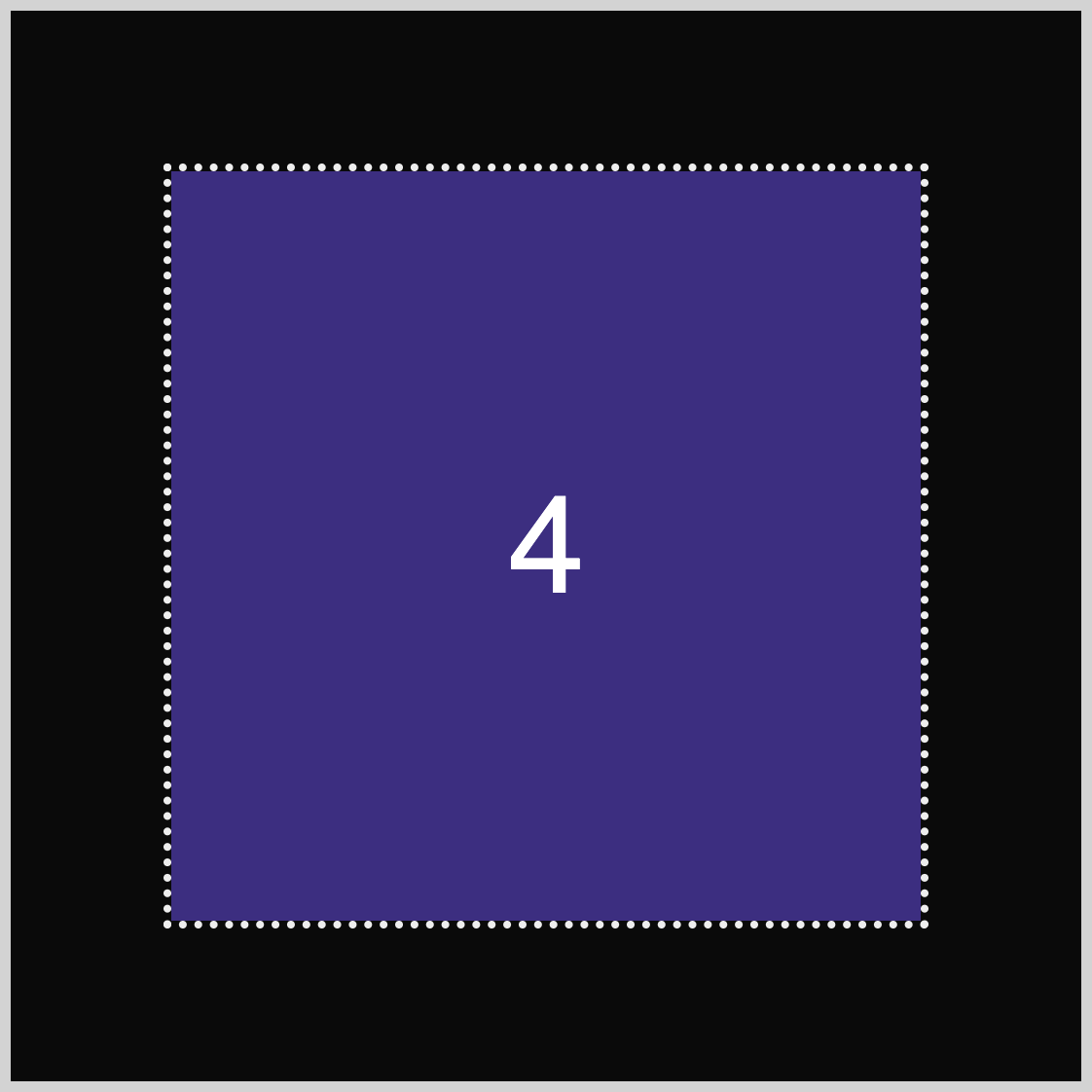
In this final section, you’ll combine scale3d()
and rotate3d()
once more to create a variation of the third example.
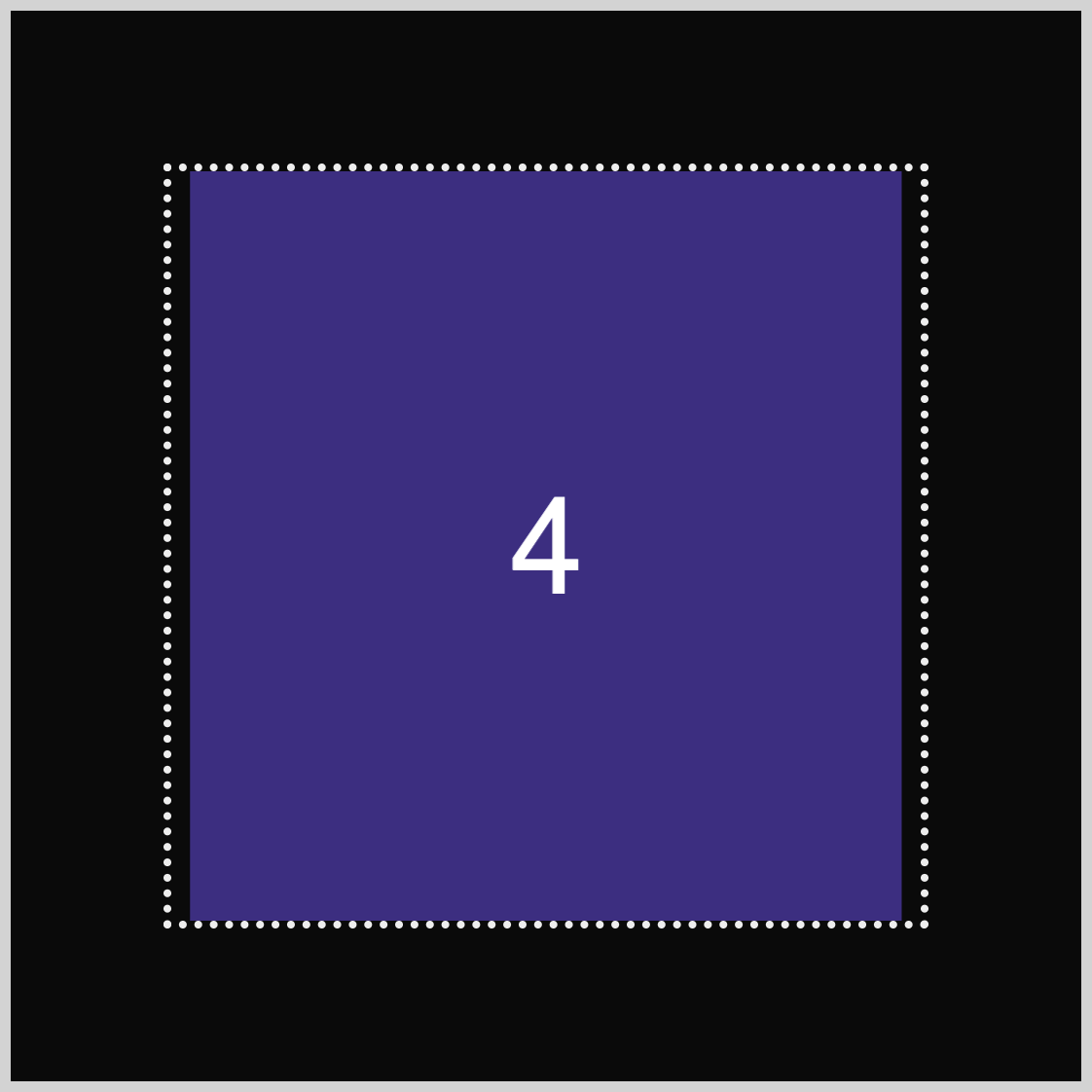
.square.four {
background: #6e4ff87f;
transform: scale3d(0.95, 0.95, 0.95) rotate3d(0, 1, 0, 60deg);
}
The background property utilizes #6e4ff87f
, a violet hue featuring 50% transparency. The values in the scale3d()
function are all set to 0.95
, reducing the square to 95% of its initial width and height. The rotate3d(0, 1, 0, 60deg)
rotates the square around its vertical (y-axis) by 60 degrees.
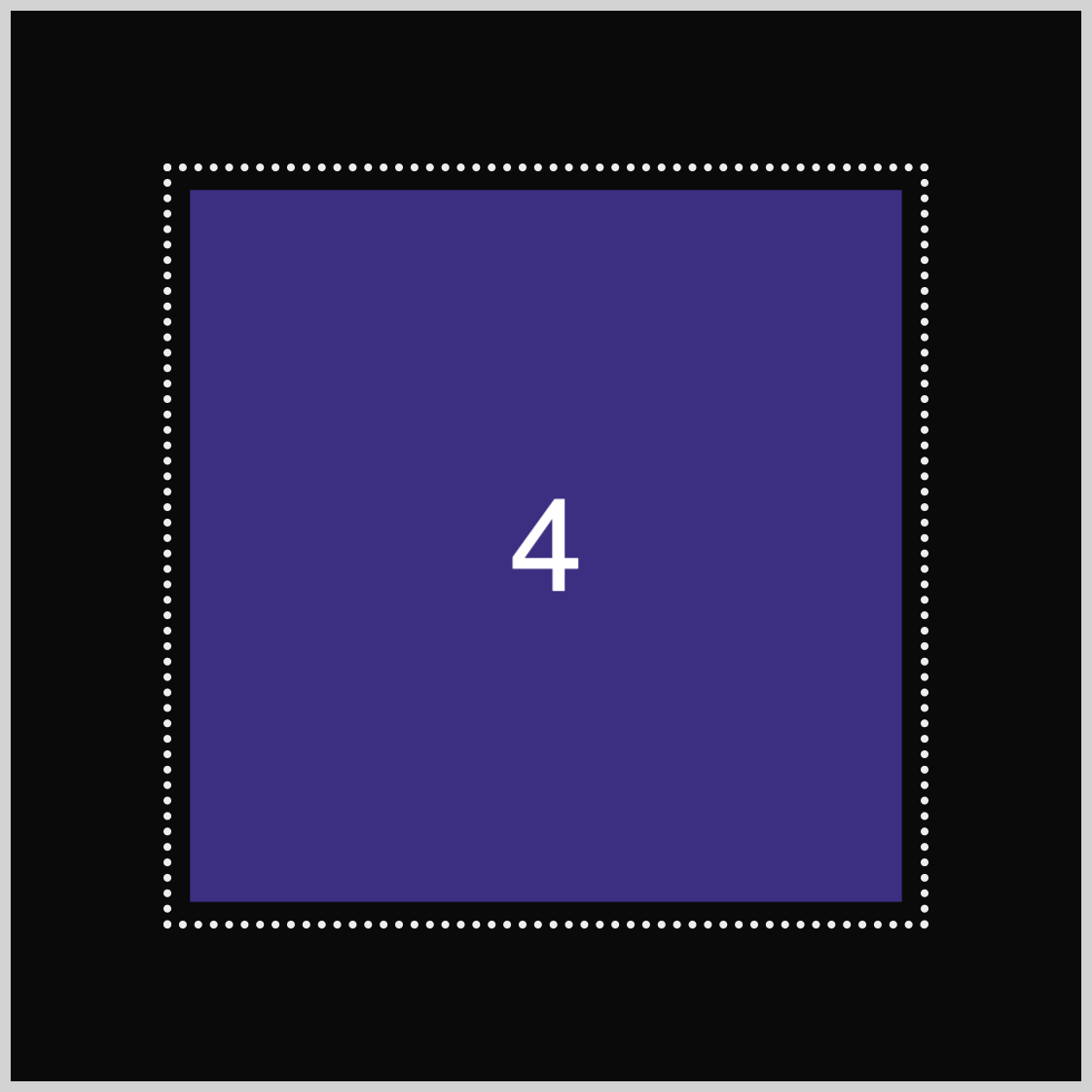
The square is reduced to 95% at this point. The rotate3d()
function has not been applied to the square yet.
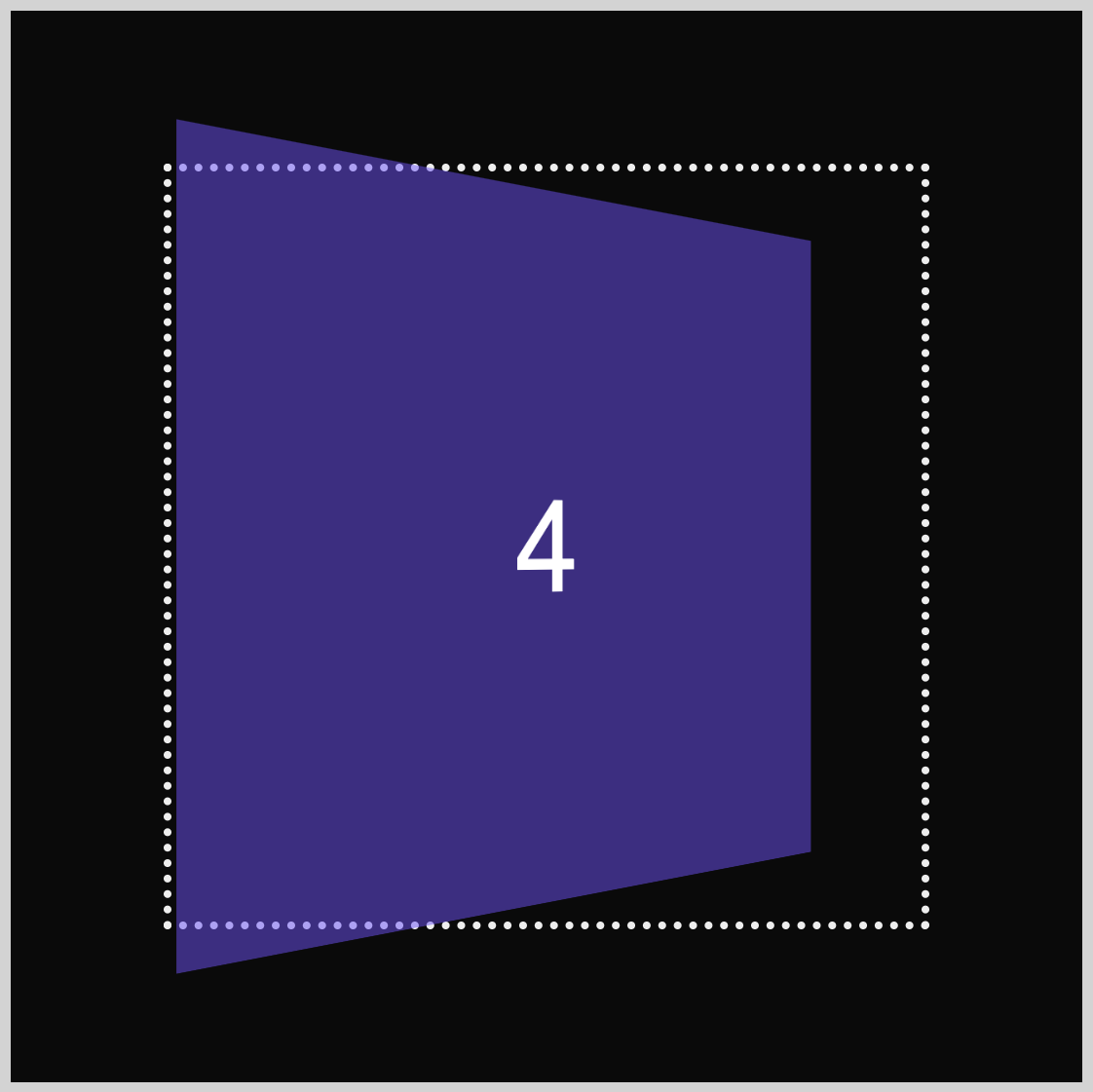
The rotate3d()
rotation angle is set to 30 degrees, tilting the square’s right side backward.
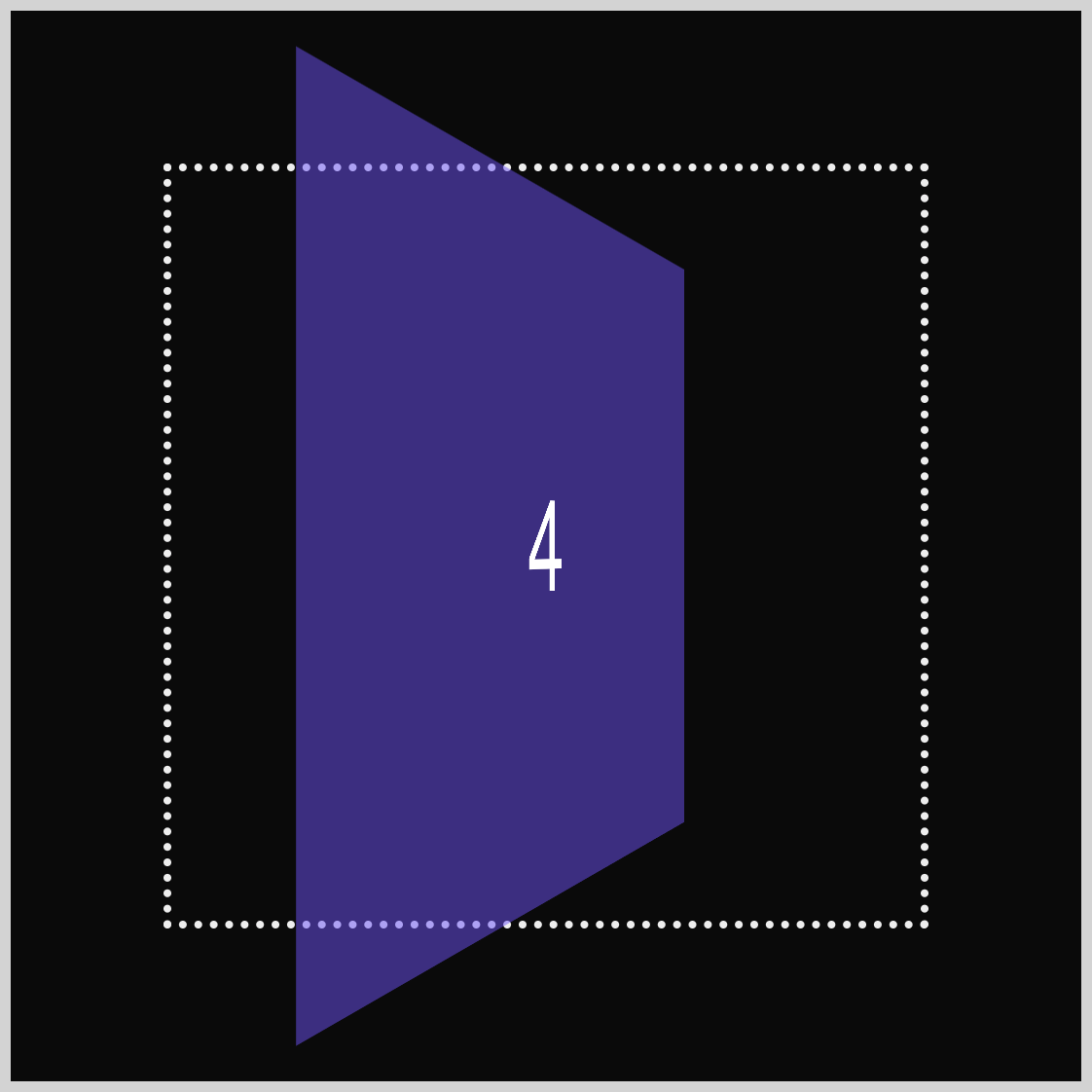
The square has reached its end shape with a rotation angle of 60 degrees.
You can see and play with the complete code on Pyxofy’s CodePen page.
See the Pen CSS Art – scale3d() with rotate3d() Transforms by Pyxofy (@pyxofy) on CodePen.
Conclusion
This article examined the CSS scale3d()
function in depth using multiple 3D transform examples. You learned how each value of the scale3d()
function affects the scale and shape of the square in three-dimensional (3D) space.
Using scale3d()
with rotate3d()
enhances your 3D options by allowing you to configure the function’s various axes and angles of rotation. What 3D objects and images will you make? Share your masterpiece with us on LinkedIn, Threads, Bluesky, Mastodon, X (Twitter) @pyxofy, or Facebook.
We hope you liked this article. Kindly share it with your network. We appreciate it.